Hello,
The answer here is to change the SmoothingMode, e.g.
Code: Select all
Random random = new Random();
private void InitializeChart()
{
tChart1.CurrentTheme = ThemeType.Opera;
tChart1.Graphics3D.SmoothingMode = SmoothingMode.HighSpeed;
Area area = new Area();
area.Color = Color.GreenYellow;
area.AreaBrush.Gradient.StartColor = Color.GreenYellow;
area.AreaBrush.Gradient.MiddleColor = Color.BlueViolet;
area.AreaBrush.Gradient.EndColor = Color.Crimson;
area.AreaBrush.Gradient.UseMiddle = true;
area.AreaBrush.Gradient.Visible = true;
area.AreaBrush.Gradient.Transparency = 20;
area.AreaBrush.Gradient.GammaCorrection = true;
area.LinePen.Visible = false;
area.AreaLines.Visible = false;
var x = 0d;
for (int i = 0; i <= 30; i++)
{
x = random.NextDouble() * 10 + x;
var y = random.NextDouble() * 5;
area.Add(x, y);
}
tChart1.Series.Add(area);
}
which gives me:
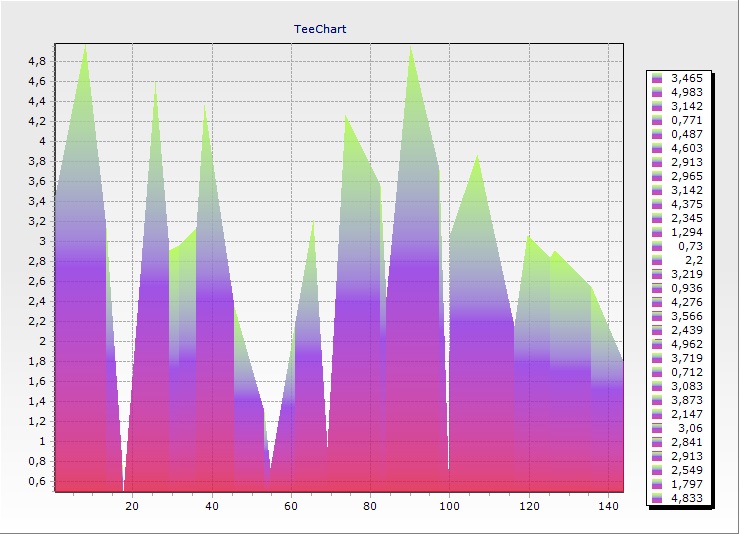
- 636083281774883694.jpg (119.01 KiB) Viewed 20016 times
FYI, you can see this effect outside of TeeChart, e.g. in a UserControl:
Code: Select all
public partial class UserControl2 : UserControl
{
public UserControl2()
{
InitializeComponent();
}
Random rnd = new Random();
protected override void OnPaint(PaintEventArgs e)
{
Func<Rectangle, Color, Color, Color, LinearGradientBrush> Gradient = (rect, startColor, middleColor, endColor) =>
{
LinearGradientBrush result = new LinearGradientBrush(rect, startColor, endColor, LinearGradientMode.Vertical);
ColorBlend blend = new ColorBlend(3);
blend.Colors[0] = startColor;
blend.Colors[1] = middleColor;
blend.Colors[2] = endColor;
blend.Positions[0] = 0;
blend.Positions[1] = 0.5F;
blend.Positions[2] = 1;
result.InterpolationColors = blend;
result.GammaCorrection = false;
return result;
};
Func<Point[], Rectangle> BoundingRect = (p) =>
{
Rectangle r = new Rectangle();
if (p.GetUpperBound(0) > 1)
{
r.X = p[0].X;
r.Width = 0;
r.Y = p[0].Y;
r.Height = 0;
for (int t = 1; t <= p.GetUpperBound(0); t++)
{
if (p[t].X < r.Left)
{
r.Width = r.Right - p[t].X;
r.X = p[t].X;
}
if (p[t].X > r.Right)
{
r.Width = r.Width + p[t].X - r.Right;
}
if (p[t].Y < r.Top)
{
r.Height = r.Bottom - p[t].Y;
r.Y = p[t].Y;
}
if (p[t].Y > r.Bottom)
{
r.Height = r.Height + p[t].Y - r.Bottom;
}
}
}
return r;
};
Graphics g = e.Graphics;
g.SmoothingMode = SmoothingMode.HighQuality;
Rectangle bounds = this.Bounds;
int count = 30;
int width = bounds.Width / count;
int trans = (int)Math.Round(255 * 0.6);
Point oldTopRight = Point.Empty;
for (int i = 0; i < count; i++)
{
int x0 = width * i;
int y0 = rnd.Next(bounds.Height);
int x1 = x0 + width;
int y1 = bounds.Height;
Point topLeft = new Point(x0, y0);
Point topRight = new Point(x1, y0);
Point bottomRight = new Point(x1, y1);
Point bottomLeft = new Point(x0, y1);
Point[] poly = new Point[] { oldTopRight == Point.Empty ? topLeft : oldTopRight, topRight, bottomRight, bottomLeft};
Rectangle rect = new Rectangle(x0, y0, width, bounds.Height - (oldTopRight == Point.Empty ? y0 : Math.Min(oldTopRight.Y, y0)));
LinearGradientBrush grad = Gradient(BoundingRect(poly), Color.FromArgb(trans, Color.GreenYellow), Color.FromArgb(trans, Color.BlueViolet), Color.FromArgb(trans, Color.Crimson));
g.FillPolygon(grad, poly);
oldTopRight = topRight;
}
}
}
use of which gives me:
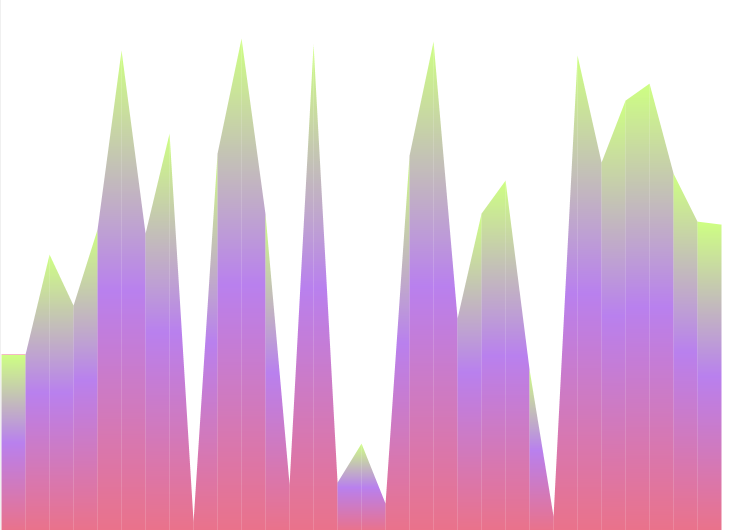
- usercontrol.PNG (69.68 KiB) Viewed 20015 times